Connecting Two BLE Devices in iOS
Jermaine Daniel
· 3 min read min read
0
0
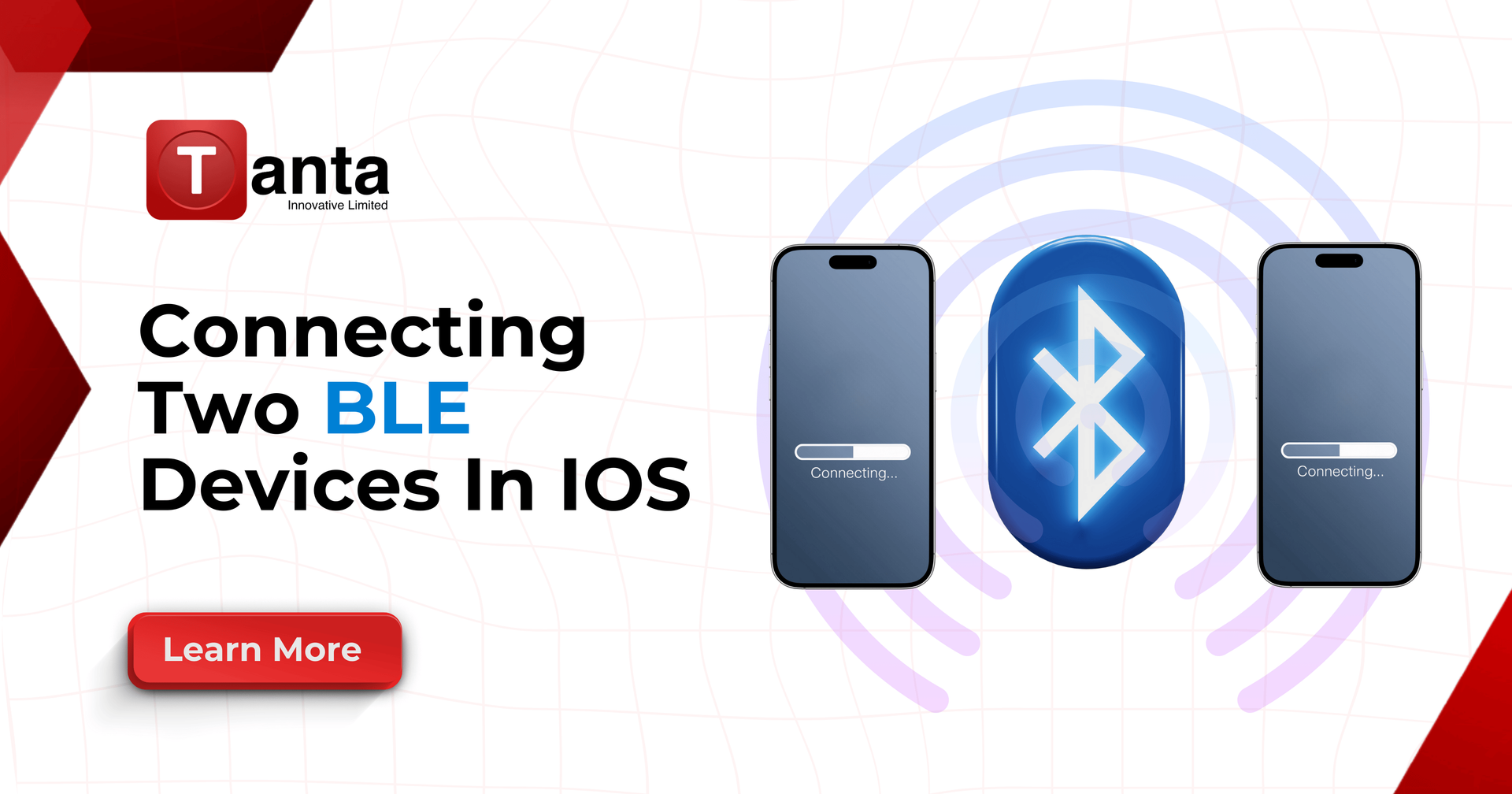
Introduction
Bluetooth Low Energy (BLE) has become a cornerstone technology in the Internet of Things (IoT) ecosystem, offering energy-efficient wireless communication for a wide range of devices. This article provides a detailed guide on implementing BLE connectivity between two devices in iOS, covering everything from basic concepts to practical implementation using Swift.
Understanding BLE Basics
Before diving into the implementation, it's crucial to understand some fundamental BLE concepts:
- Central and Peripheral Roles:
- Central devices (usually smartphones or tablets) scan for and connect to peripherals.
- Peripheral devices (often sensors or IoT devices) advertise their presence and wait for connections.
- Services and Characteristics:
- Services are collections of data and associated behaviors.
- Characteristics are the actual data points within a service.
- GATT (Generic Attribute Profile):
- Defines how data is organized and exchanged over BLE.
Implementing BLE in iOS
iOS provides the CoreBluetooth framework for working with BLE. Here's a step-by-step guide to implementing a BLE manager that can connect to two devices:
Step 1: Setting Up the BluetoothViewModel
First, we'll create a BluetoothViewModel class that will manage our BLE connections:
Step 2: Implementing Scanning for Devices
To discover nearby BLE devices, we need to implement scanning:
Step 3: Connecting to Devices
Now, let's implement methods to connect to two separate devices:
Step 4: Handling Disconnections
It's important to handle disconnections gracefully:
Step 5: Discovering Services and Characteristics
Once connected, we need to discover services and characteristics:
Step 6: Exchanging Data Between Devices
Finally, let's implement a method to exchange data between the connected devices:
Using the BluetoothViewModel
To use this implementation in your app:
- Create an instance of BluetoothViewModel or use the shared instance.
- Call startScanning() to begin discovering nearby devices.
- Use the devices array to present available devices to the user.
- Call connectToPeripheral1(peripheral:) and connectToPeripheral2(peripheral:) to connect to the chosen devices.
- Monitor isConnectedToDevice1 and isConnectedToDevice2 to know when both devices are connected.
- Use exchangeDataBetweenDevices(data:) to send data between the connected devices.
Considerations and Best Practices
- Error Handling: Implement robust error handling for various Bluetooth-related scenarios.
- Power Management: Be mindful of battery usage. Stop scanning when not needed.
- User Experience: Provide clear feedback to users about the connection status and any issues.
- Security: Implement appropriate security measures, especially when dealing with sensitive data.
- Testing: Thoroughly test with various device combinations and in different scenarios.
Conclusion
Implementing BLE connectivity between two devices in iOS requires a good understanding of the CoreBluetooth framework and careful management of device connections. The BluetoothViewModel class provided in this article offers a solid foundation for building BLE-enabled iOS applications that can connect to and communicate with multiple devices simultaneously.
As BLE technology continues to evolve, staying updated with the latest iOS capabilities and Bluetooth specifications will be crucial for developing robust and efficient IoT applications.
GitHUB Link -
https://github.com/bigjermaine/Bluetooth-Connectivity-
Edit
Edit
Remove
Remove
https://github.com/bigjermaine/Bluetooth-Connectivity-
IOS developer
More from Jermaine Daniel

Enhancing SwiftUI Previews for UIKit Components
Swift...


Mastering MusicKit Integration in iOS Applications
Learn how to integrate Apple Music features into your iOS app using MusicKit. Access a vast music library, enable seamle...


Introducing Swift 6 with Enhanced Concurrency and Performance
Swift 6 is here with groundbreaking updates, from improved concurrency and error handling to C++ interoperability and em...


Setting Up Notifications in Xcode Cloud
In the fast-paced world of iOS development, staying informed about your build statuses is crucial. Xcode Cloud offers a ...

Related Articles
Discover more insights and stories from our collection of articles
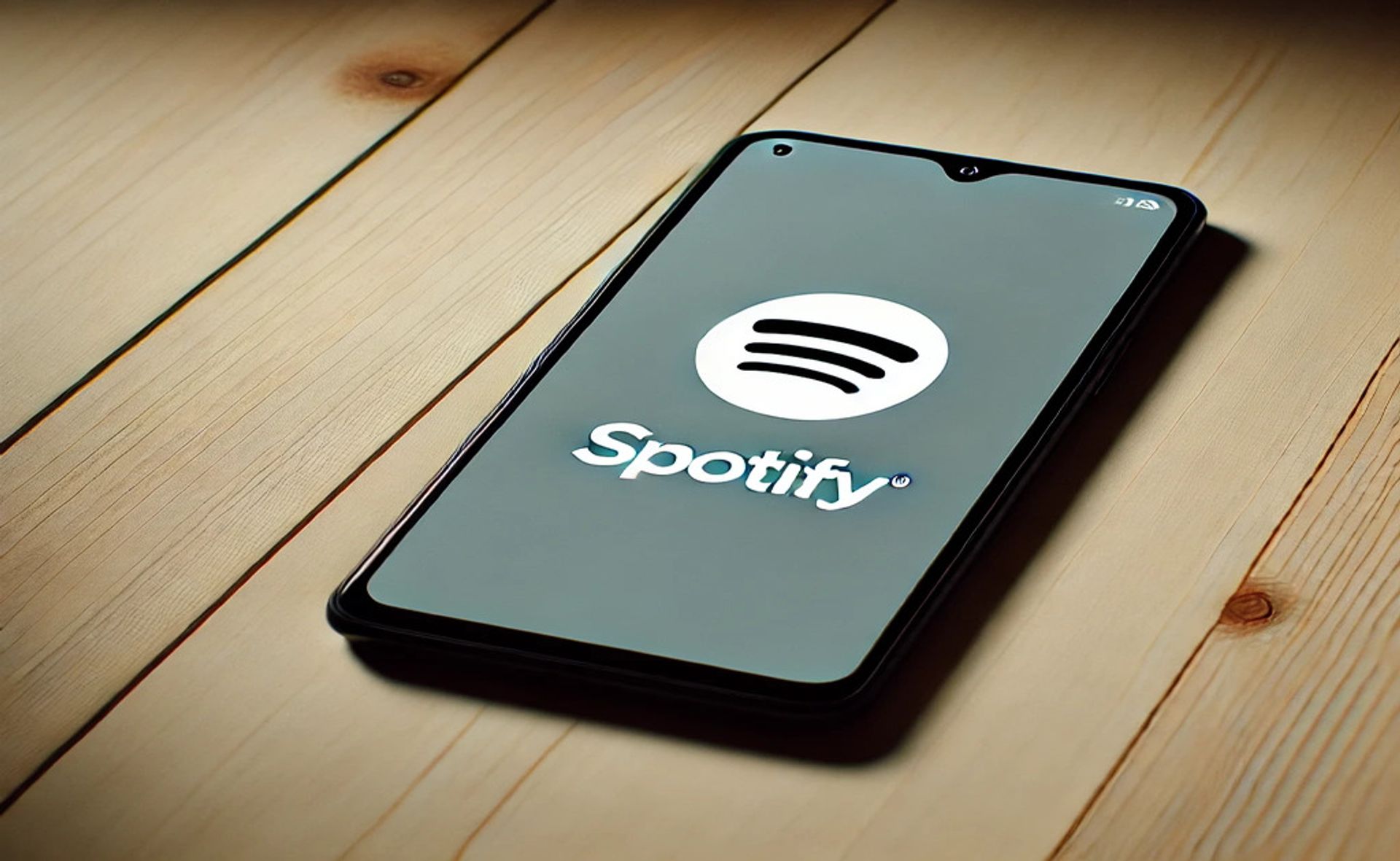
Integrating Spotify in Android Apps: Web API + SDK Tutorial 2025
Learn how to integrate Spotify's Web API and SDK into your Android app to add music streaming, user data, and playlist features—perfect for unique portfolio projects.
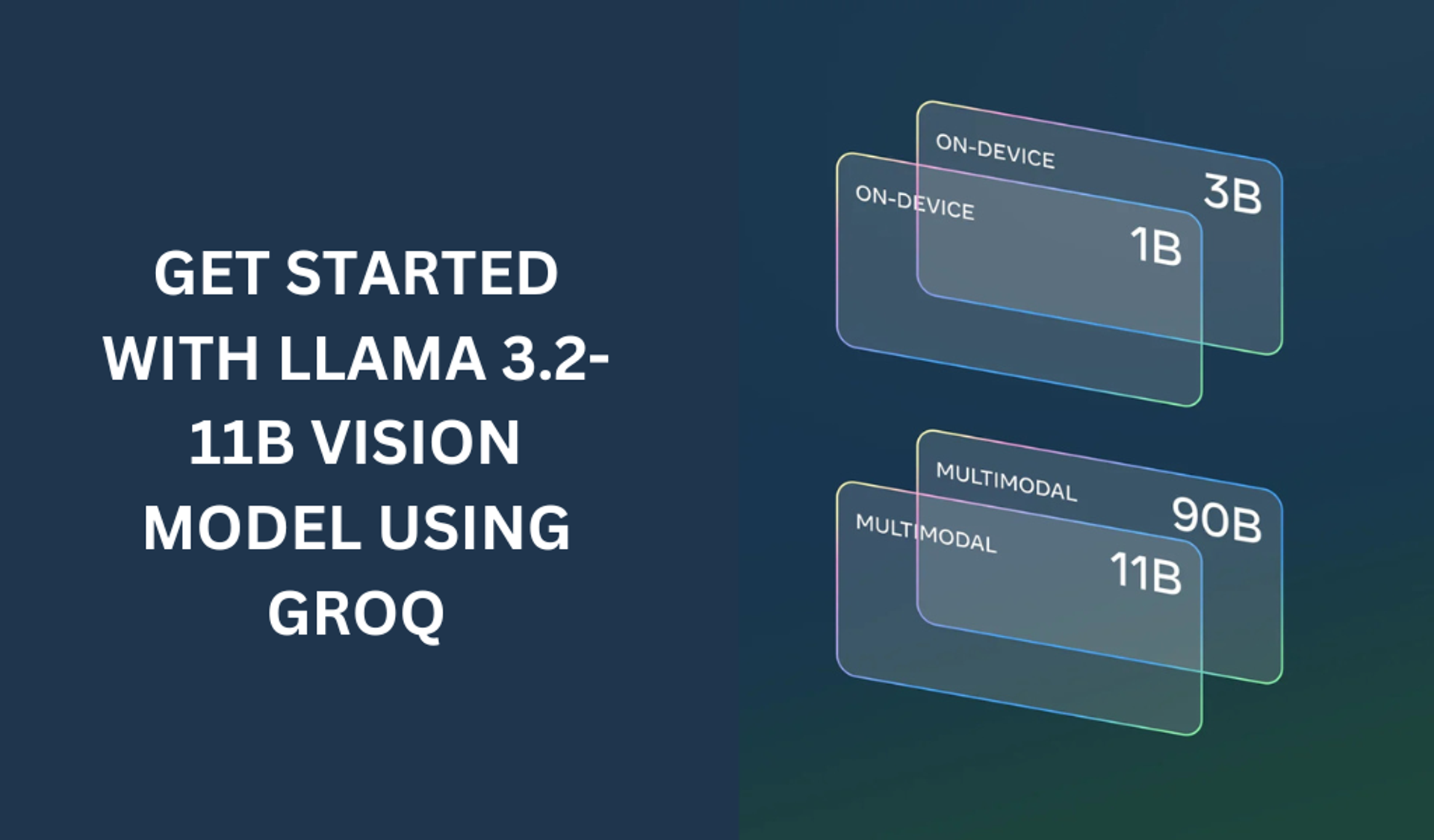
Getting started with the LLAMA 3.2-11B with groq
The LLAMA 3.2-11B model is a very powerful model that can perform both text and vision tasks.
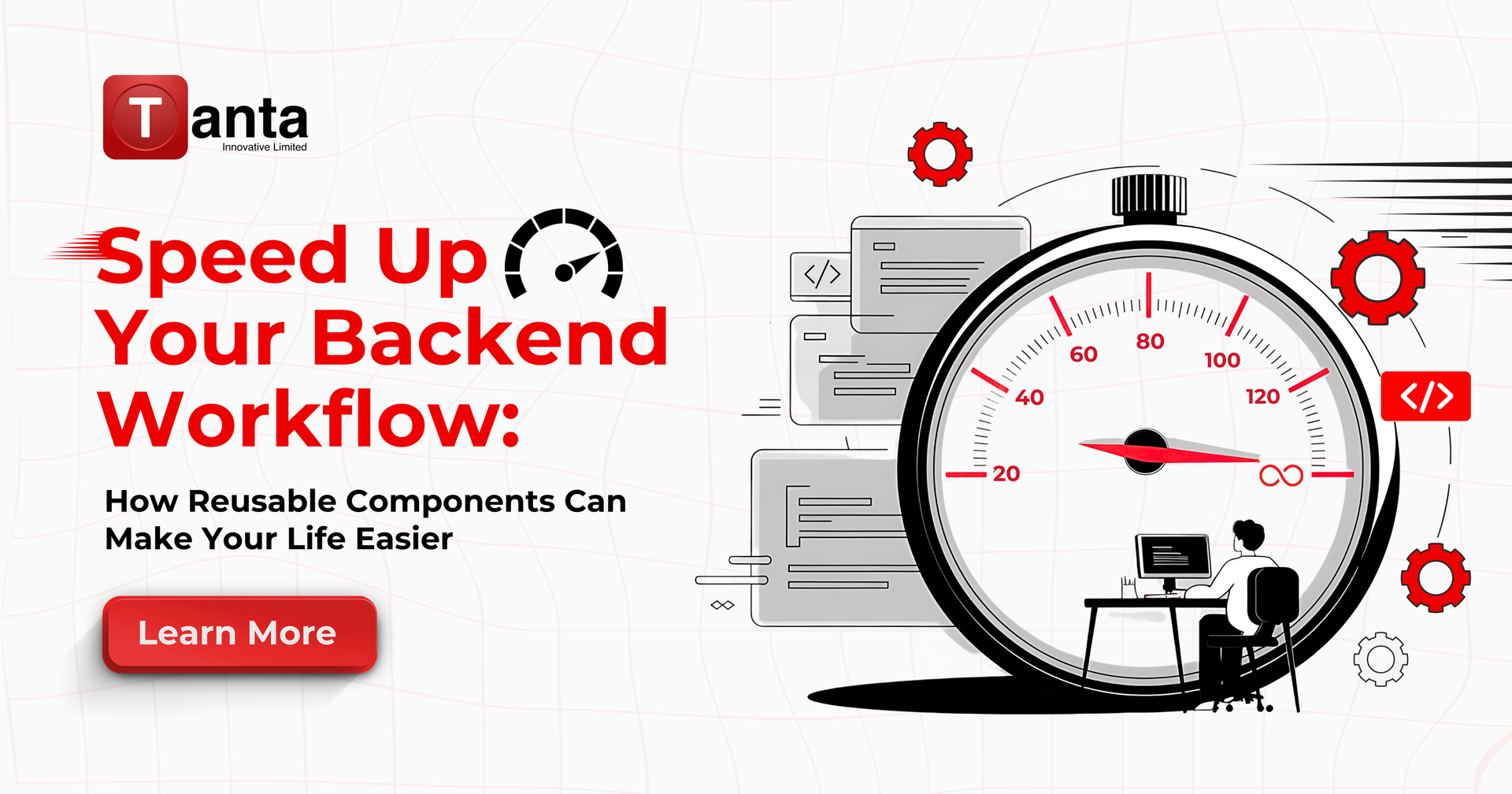
Streamline Your Backend Workflow with Reusable Components
Learn how backend developers can save time by creating reusable components for common tasks, streamlining project setups, improving consistency, and boosting productivity.