Integrating Spotify in Android Apps: A Developer's Guide to the Spotify Web API and SDK
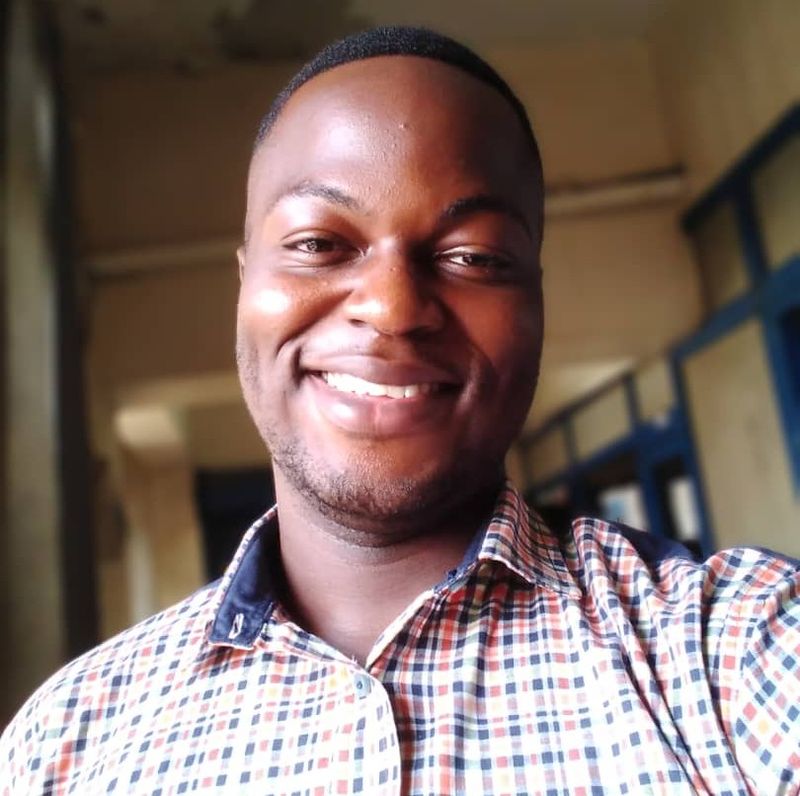
Olisemeka Nwaeme
· 2 min read
0
0
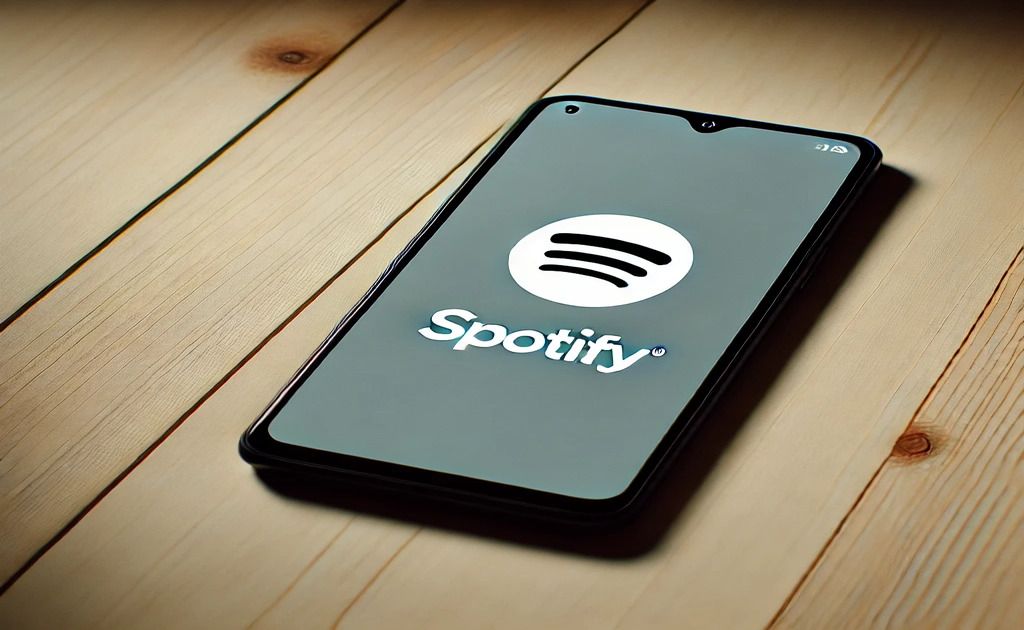
Introduction
Integrating Spotify’s Web API and Android SDK opens exciting possibilities for adding music features, custom playlists, and extensive music data into Android applications. This guide explores how these tools can transform projects, enhancing user engagement with streaming capabilities and data-driven features. Whether you’re an experienced developer or a new developer seeking a standout portfolio project, Spotify’s tools offer resources to enrich your app with the power of music. Let’s dive into how you can bring Spotify’s world of music right into your app.
Prerequisites
Before diving into Spotify integration on Android, ensure your development environment meets the following requirements
- Android Studio
- Android API Level 21+
- A Spotify account
Set Up Your App on the Spotify Developer Dashboard
- Create Your Spotify App:
Go to Spotify Developer Dashboard and fill out the form to create your app. One required field is the Redirect URI, which you’ll need to set up for your Android app. - Define Redirect URI in Your Manifest:
In your AndroidManifest.xml file, add an intent filter within the <activity> tag of your MainActivity (or whichever activity you created for Spotify integration):
1
2<intent-filter>
3 <action android:name="android.intent.action.VIEW" />
4 <category android:name="android.intent.category.DEFAULT" />
5 <category android:name="android.intent.category.BROWSABLE" />
6 <data
7 android:host="callback"
8 android:scheme="mycustomapp" />
9</intent-filter>
10
Replace "mycustomapp" with your app name.
- Store Redirect URI as a Constant:
Define the following URI as a constant in your app: "appname://mycustomapp". This will be needed later. Copy this URI and paste it into the Redirect URI field in the Spotify Developer Dashboard. - Configure Package Name and SHA1 Fingerprint:
After clicking Create, navigate to Settings > Edit to add your app's package name and SHA1 fingerprint. This is crucial, as missing this step could cause errors during Spotify integration.
To retrieve your SHA1 fingerprint, open the terminal in Android Studio and run
./gradlew signingReport
If this doesn’t work, search online for instructions specific to your OS. After adding both the package name and SHA1 fingerprint, click Save to complete the setup.
Add the Spotify SDK to Your App
To leverage Spotify's playback features in your app, you’ll need to integrate the Spotify Android SDK. This SDK enables your app to control playback on the Spotify Android app. Ensure that the Spotify Android app is installed on the device.
- Download the SDK:
Download the .aar file from this link. - Move the SDK to Your Project:
Place the downloaded .aar file in the root of your Android project directory, or ideally within a libs folder for better organization. - Add the SDK as a Dependency in Android Studio:
- Open Android Studio and go to File > Project Structure > Dependencies.
- Click the + icon under All Dependencies.
- Select JAR/AAR Dependency and enter the path to the .aar file (e.g., ../spotify-app-remote-release-0.8.0.aar if you placed it in the root directory).
- Click OK to add the dependency.
Perform Spotify User Authorization
To access Spotify user data, your app must be authorized by Spotify. Scopes—an array of strings specifying the level of access to a user’s information—are essential to this authorization. You can find detailed scope requirements in the Spotify Web API documentation.
- Retrieve Your Client ID:
Go to your app on the Spotify Developer Dashboard, copy your client ID, and save it as a constant in your app. You’ll use this ID to request user authorization. - Create an Authorization Request:
Use the following code snippet to build an authorization request. - Open the Spotify Login Activity:
Launch Spotify’s login screen using the authorization request:
1
2AuthorizationClient.openLoginActivity(
3 this,
4 SPOTIFY_AUTH_REQUEST_CODE, // Assign any unique integer value
5 authRequest
6)
7
- Retrieve the Access Token:
In onActivityResult, capture the access token, which will be needed for making requests to the Spotify Web API. Be sure to store this token securely, as it provides access to the authorized user’s data.
1override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
2 super.onActivityResult(requestCode, resultCode, data)
3 if (requestCode == SPOTIFY_AUTH_REQUEST_CODE) {
4 val authResponse = AuthorizationClient.getResponse(resultCode, data)
5 when (authResponse.type) {
6 AuthorizationResponse.Type.TOKEN -> {
7 viewModel.spotifyAuthTokenResponse.value = authResponse.accessToken
8 }
9
10 AuthorizationResponse.Type.ERROR -> {
11 Toast.makeText(
12 this,
13 "Error occurred: ${authResponse.error}",
14 Toast.LENGTH_SHORT
15 ).show()
16 }
17
18 else -> {
19 //Nothing
20 }
21 }
22 }
23}
Access the Spotify Web API Endpoints
Visit the Spotify Web API documentation for comprehensive details on each available API endpoint. With these endpoints, you can fetch user data, favorite artists, recently played tracks, create new playlists, and much more.
Play a Track or Playlist
To play a specific track or playlist retrieved from the Spotify Web API, use the SpotifyAppRemote object provided by the Spotify Android SDK. Follow these steps to establish a connection and initiate playback:
- Define Connection Variables:
Set up a variable for SpotifyAppRemote and define the connection parameters using your Spotify client ID and redirect URI.
1
2private var spotifyAppRemote: SpotifyAppRemote? = null
3private val connectionParams = ConnectionParams.Builder(SPOTIFY_CLIENT_ID)
4 .setRedirectUri(SPOTIFY_REDIRECT_URI)
5 .showAuthView(true)
6 .build()
7
- Connect to Spotify:
Call SpotifyAppRemote.connect() with the required arguments, including a listener interface with callbacks for connection success or failure. On a successful connection, initialize spotifyAppRemote with the appRemote instance from the onConnected callback.
1
2SpotifyAppRemote.connect(
3 this,
4 connectionParams,
5 object : Connector.ConnectionListener {
6 override fun onConnected(appRemote: SpotifyAppRemote) {
7 spotifyAppRemote = appRemote
8 onSpotifyConnected() // Call this when the connection is successful
9 }
10
11 override fun onFailure(throwable: Throwable) {
12 Log.e("MyActivity", "Connection failed: ${throwable.message}")
13 }
14 }
15)
16
- Initiate Playback:
In onSpotifyConnected(), use playerApi.play() with the URI of the track or playlist obtained from the Web API response.
1private fun onSpotifyConnected() {
2 spotifyAppRemote?.playerApi?.play("spotify:playlist:37i9dQZF1DX2sUQwD7tbmL")
3 // Replace with the URI of your desired track or playlist
4}
Conclusion
Integrating Spotify’s SDK and Web API into your Android app opens up a world of music-driven experiences for your users, from seamless playback control to personalized data insights. With these tools, your app can bring users closer to their favorite artists, playlists, and tracks, all while leveraging Spotify's powerful ecosystem. Now it’s time to bring your musical vision to life—happy coding, and may the music be with you!
Olise is a product-oriented Software Engineer specializing in Android development. As the Android Development Lead at Tanta Innovative, he’s dedicated to creating impactful mobile solutions that elevate client success and drive business efficiency. Beyond coding, Olise is a passionate Arsenal FC fan and anime lover. He values community and enjoys connecting with fellow developers at meetups.