Enhancing SwiftUI Previews for UIKit Components
Jermaine Daniel
· 3 min read min read
0
0
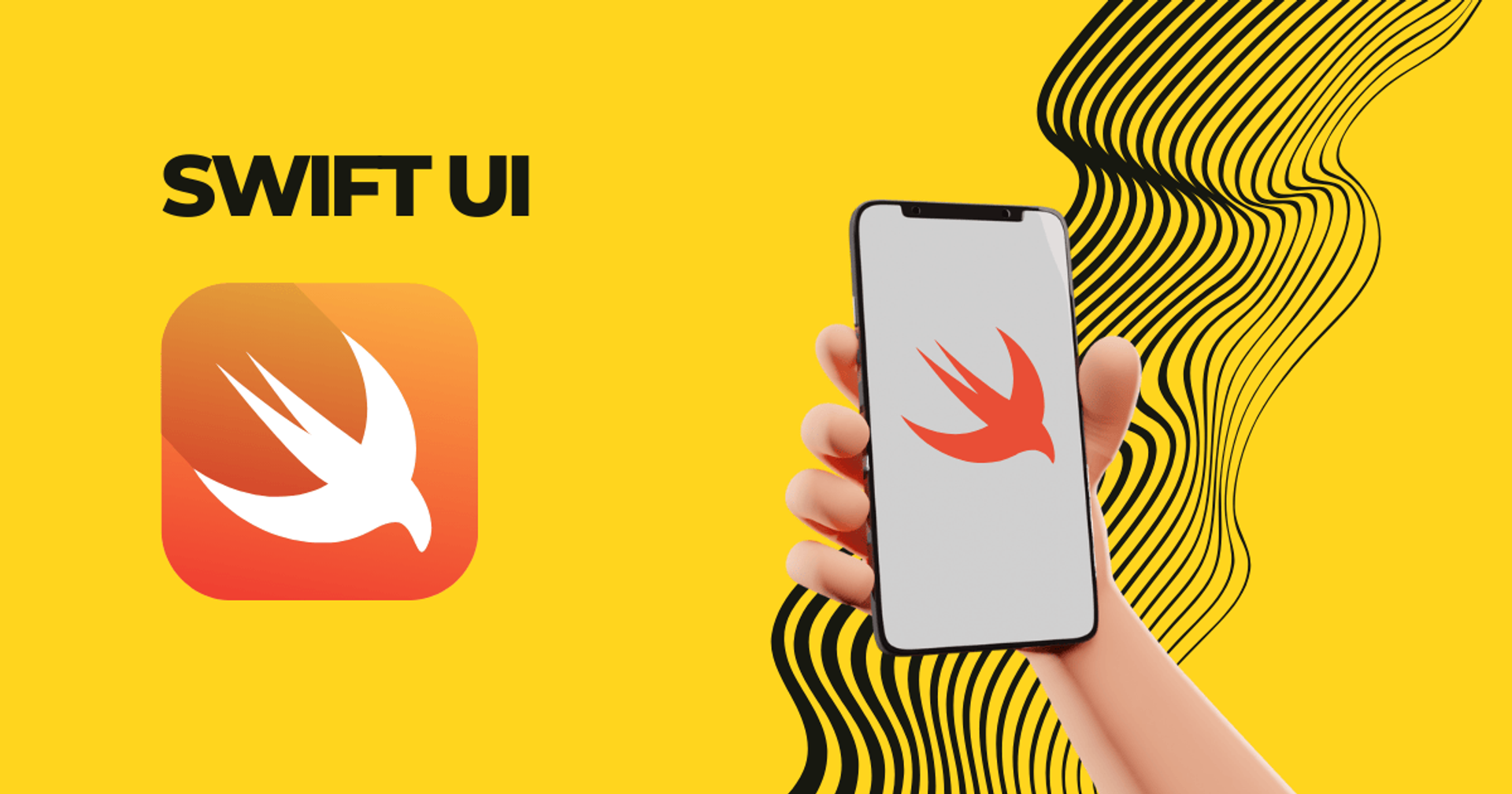
IOS developer
More from Jermaine Daniel

Mastering MusicKit Integration in iOS Applications
Learn how to integrate Apple Music features into your iOS app using MusicKit. Access a vast music library, enable seamle...


Introducing Swift 6 with Enhanced Concurrency and Performance
Swift 6 is here with groundbreaking updates, from improved concurrency and error handling to C++ interoperability and em...


Setting Up Notifications in Xcode Cloud
In the fast-paced world of iOS development, staying informed about your build statuses is crucial. Xcode Cloud offers a ...


Leveraging Free Firebase Analytics for Mobile App Business Decisions
Review where your new users are coming from (e.g., organic search, ads, referrals). Compare the number of new users fro...

Related Articles
Discover more insights and stories from our collection of articles
-1920x1008.png)
Boost your business growth with these apps
Tanta Innovatives has established itself as a key partner for businesses seeking to enhance their operations and achieve sustainable growth. Through custom software solutions, advanced cybersecurity measures, and effective CRM implementations, Tanta Innovatives has empowered businesses across various industries.
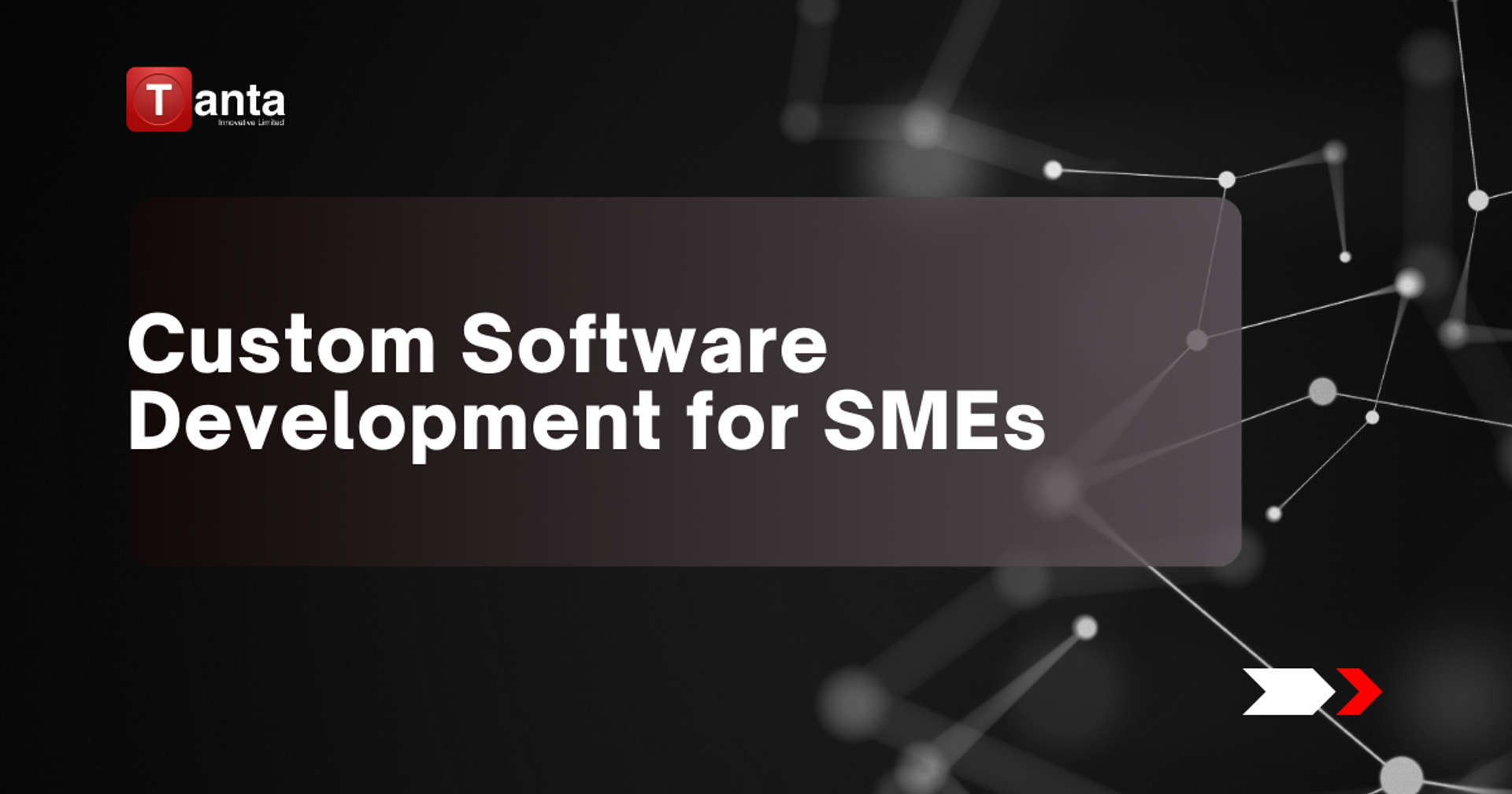
Empowering Nigerian SMEs with Custom Software Development for a Competitive Future
Tanta Innovative Company specializes in custom software development for Nigerian SMEs. Our tailored solutions help businesses streamline operations, scale efficiently, and gain a competitive edge. With seamless integration, expert consultation, and ongoing support, we empower SMEs to grow through technology. Let us craft software that works for you, not the other way around.