Mastering MusicKit Integration in iOS Applications
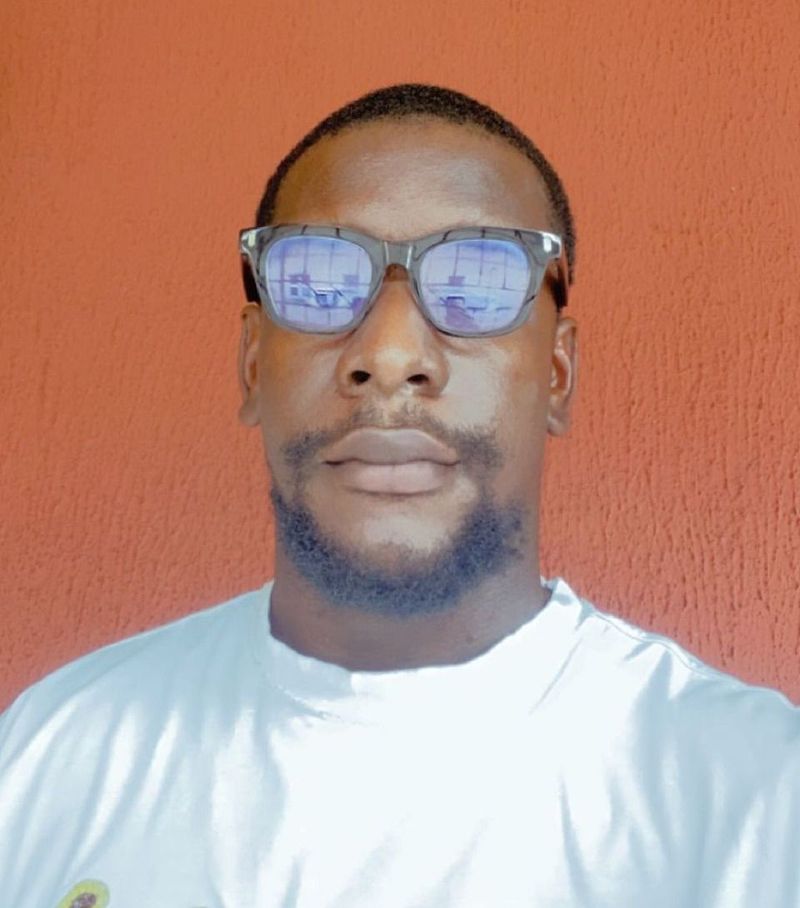
Jermaine Daniel
· 2 min read
0
0
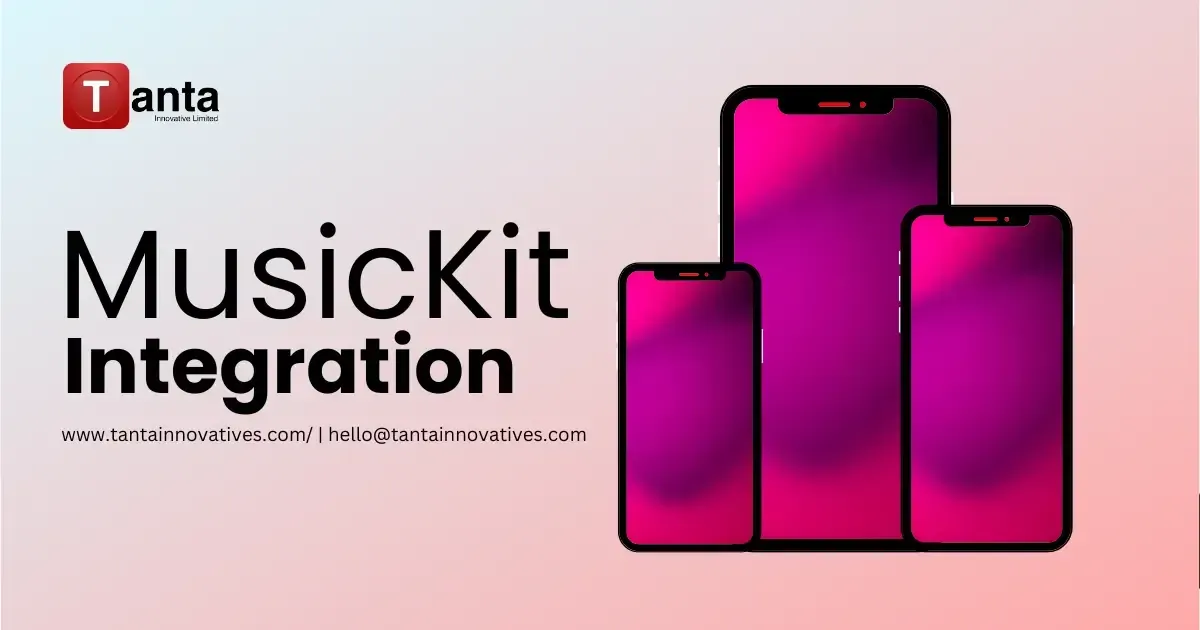
Introduction to MusicKit
MusicKit is a powerful framework provided by Apple that enables developers to seamlessly integrate Apple Music features into their iOS applications. By leveraging MusicKit, you can create rich, engaging music experiences that tap into Apple's vast library of over 90 million songs..
Key Advantages of MusicKit Integration:
- Access to Apple Music's extensive catalog
- Seamless playback of Apple Music content
- Creation of personalized music experiences
- Smooth integration with users' existing Apple Music accounts
- Advanced audio features like Dolby Atmos support
Project Setup and Configuration
Before diving into MusicKit integration, ensure your development environment meets the following requirements:
- Xcode 12 or later
- iOS 14.0+ as the minimum deployment target
- Active Apple Developer account
Configuring Your Xcode Project:
- Open your project in Xcode.
- Navigate to the project navigator and select your target.
- In the "Signing & Capabilities" tab, add the "Music Kit" capability.
- Update your `Info.plist` with the following keys:
1<key>NSAppleMusicUsageDescription</key>
2<string>This app requires access to Apple Music to provide personalized music recommendations and playback.</string>
3
4<key>NSMicrophoneUsageDescription</key>
5<string>Microphone access is needed for music recognition features.</string>
6
7<key>kTCCServiceMediaLibrary</key>
8<string>Access to your media library allows us to integrate your personal music collection.</string>
Authentication and Authorization
To utilize MusicKit effectively, you need to set up Apple Music API access:
- Visit the Apple Developer portal and create a new "MusicKit" identifier.
- Generate a private key associated with your identifier.
- Use the private key to create a developer token for API requests.
Core MusicKit Integration
1Importing MusicKit
Start by importing MusicKit in your Swift files:
1import MusicKit
Requesting Authorization
Implement a function to request MusicKit authorization:
1func requestMusicAuthorization() async {
2 let status = await MusicAuthorization.request()
3 switch status {
4 case .authorized:
5 print("MusicKit authorization granted")
6 case .denied:
7 print("MusicKit authorization denied")
8 case .restricted:
9 print("MusicKit is restricted")
10 case .notDetermined:
11 print("MusicKit authorization not determined")
12 @unknown default:
13 print("Unknown MusicKit authorization status")
14 }
15}
Creating a Song Model
Define a Song struct to represent music tracks:
1struct Item: Identifiable {
2 let id = UUID()
3 let name: String
4 let artist:String
5 let imageUrl:URL?
6 let musicItem:Song
7}
Advanced MusicKit Features
Fetching Music Data
Implement functions to fetch various types of music data:
1// MARK: - Search Functions
2private func createBasicMusicSearchRequest(term: String = "Happy") -> MusicCatalogSearchRequest {
3 var request = MusicCatalogSearchRequest(term: term, types: [Song.self])
4 request.limit = 25
5 return request
6}
7// MARK: - Charts Functions
8private func createGlobalTopChartsRequest() -> MusicCatalogChartsRequest {
9 var request = MusicCatalogChartsRequest(kinds: [.dailyGlobalTop], types: [Song.self])
10 request.limit = 25
11 return request
12}
13private func createMostPlayedChartsRequest() -> MusicCatalogChartsRequest {
14 var request = MusicCatalogChartsRequest(kinds: [.mostPlayed], types: [Song.self])
15 request.limit = 25
16 return request
17}
18private func createCityTopChartsRequest() -> MusicCatalogChartsRequest {
19 var request = MusicCatalogChartsRequest(kinds: [.cityTop], types: [Album.self])
20 request.limit = 25
21 return request
22}
23private func createMusicSearchSuggestionsRequest(term: String = "Happy") -> MusicCatalogSearchSuggestionsRequest {
24 var request = MusicCatalogSearchSuggestionsRequest(term: term, includingTopResultsOfTypes: [Album.self])
25 request.limit = 25
26 return request
27}
These functions provide a convenient way to generate requests for various music-related data, including search results, suggestions, and chart rankings. By utilizing these requests, you can build a feature-rich music app that offers users theability to discover new music, explore trending songs and albums, and find suggestions based on their search terms. Each function is designed to set up the appropriate request type and constraints, ensuring efficient and relevant data retrieval.
Handling Dolby Atmos Information
In a music player app, you might want to indicate when a track or the current audio session is utilizing Dolby Atmos technology. Dolby Atmos is a surround sound technology that provides a more immersive listening experience compared to standard stereo audio. In MusicKit, you can check if the current track or the music player state is set to Dolby Atmos and display this information to users.
Here’s how you might handle this in SwiftUI:
To display Dolby Atmos information for tracks:
1struct ApplePlaybackControlView: View {
2 @ObservedObject var musicPlayerViewModel: ApplePlayeMusicViewModel
3 @ObservedObject var musicPlayerState = ApplicationMusicPlayer.shared.state var body: some View {
4 HStack {
5 AsyncImage(url: musicPlayerViewModel.currentSong?.artwork?.url(width: 60, height: 60)) { image in
6 image.resizable()
7 } placeholder: {
8 Color.gray
9 }
10 .frame(width: 60, height: 60)
11 .cornerRadius(8)
12 VStack(alignment: .leading) {
13 Text(musicPlayerViewModel.currentSong?.title ?? "")
14 .font(.headline)
15 Text(musicPlayerViewModel.currentSong?.artistName ?? "")
16 .font(.subheadline)
17 .foregroundColor(.gray)
18 if musicPlayerState.audioVariant == .dolbyAtmos {
19 Text("dolby")
20 }
21 }
22 Spacer()
23 Button(action: {
24 Task{
25 await musicPlayerViewModel.togglePlayPause()
26 }
27 }) {
28 Image(systemName: musicPlayerViewModel.isPlaying ? "pause.circle.fill" : "play.circle.fill")
29 .resizable()
30 .frame(width: 44, height: 44)
31 .foregroundColor(.blue)
32 }
33 }
34 .padding()
35 .background(Color.gray.opacity(0.1))
36 }
37}
Create a ViewModel to manage MusicKit interact
MusicKit Integration with ApplePlayeMusicViewModel
When discussing the ApplePlayeMusicViewModel class in the context of MusicKit, it's essential to understand how MusicKit integrates with the ApplicationMusicPlayer to manage music playback. Here’s a detailed explanation of how this ViewModel utilizes MusicKit to control music playback
MusicKit is a powerful framework provided by Apple that allows developers to access and control Apple Music content programmatically. The ApplePlayeMusicViewModel class leverages MusicKit's ApplicationMusicPlayer to handle music playback. Let's break down how this
1class ApplePlayeMusicViewModel: ObservableObject {
2 private let musicPlayer = ApplicationMusicPlayer.shared
3 @Published var currentSong: Song?
4 @Published var isPlaying: Bool = false
5 func playSong(_ song: Song) async throws {
6 let musicItem = try await MusicCatalog.search(for: song.title, types: [Song.self]).songs.first
7 if let musicItem = musicItem {
8 try await musicPlayer.play(musicItem)
9 currentSong = Song(from: musicItem)
10 isPlaying = true
11 }
12 }
13 func pauseMusic() {
14 musicPlayer.pause()
15 isPlaying = false
16 }
17 func resumeMusic() {
18 musicPlayer.play()
19 isPlaying = true
20 }
21}
This enhanced ApplePlayeMusicViewModel provides a robust interface for controlling music playback and managing the app's music state.
Conclusion
By integrating MusicKit into your iOS application, you're opening up a world of possibilities for creating rich, immersive music experiences. Remember to test your implementation on physical devices for the best results, and always consider the user experience when designing your music-related features.Happy coding, and may your app resonate with music lovers everywhere!
IOS developer
More from Jermaine Daniel
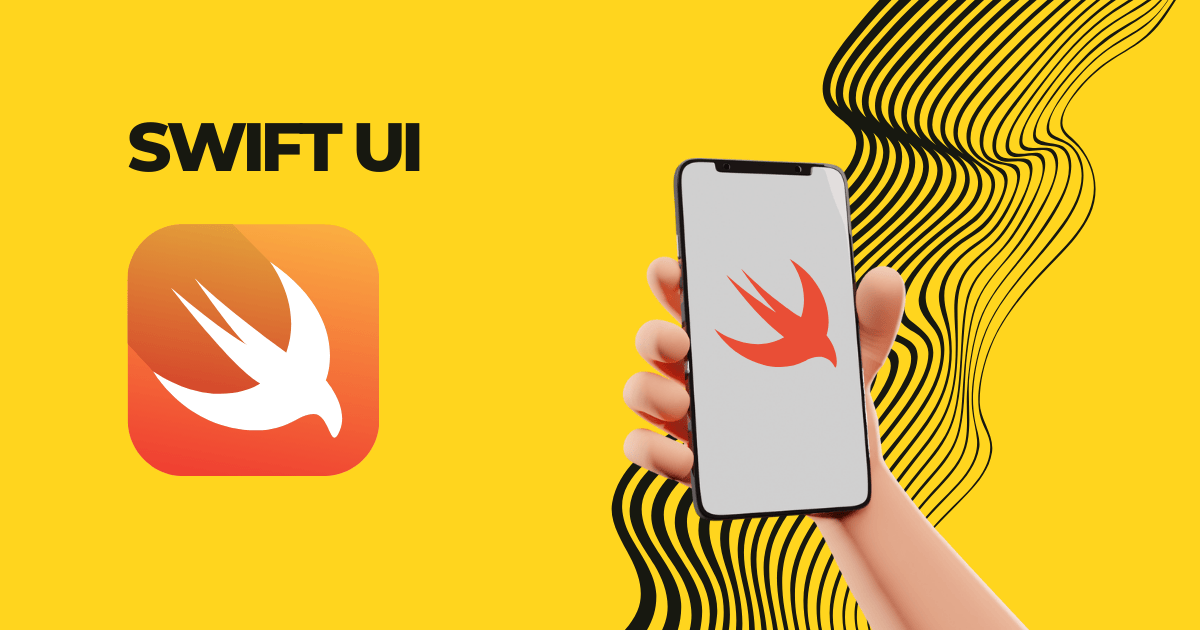
Enhancing SwiftUI Previews for UIKit Components
Swift...
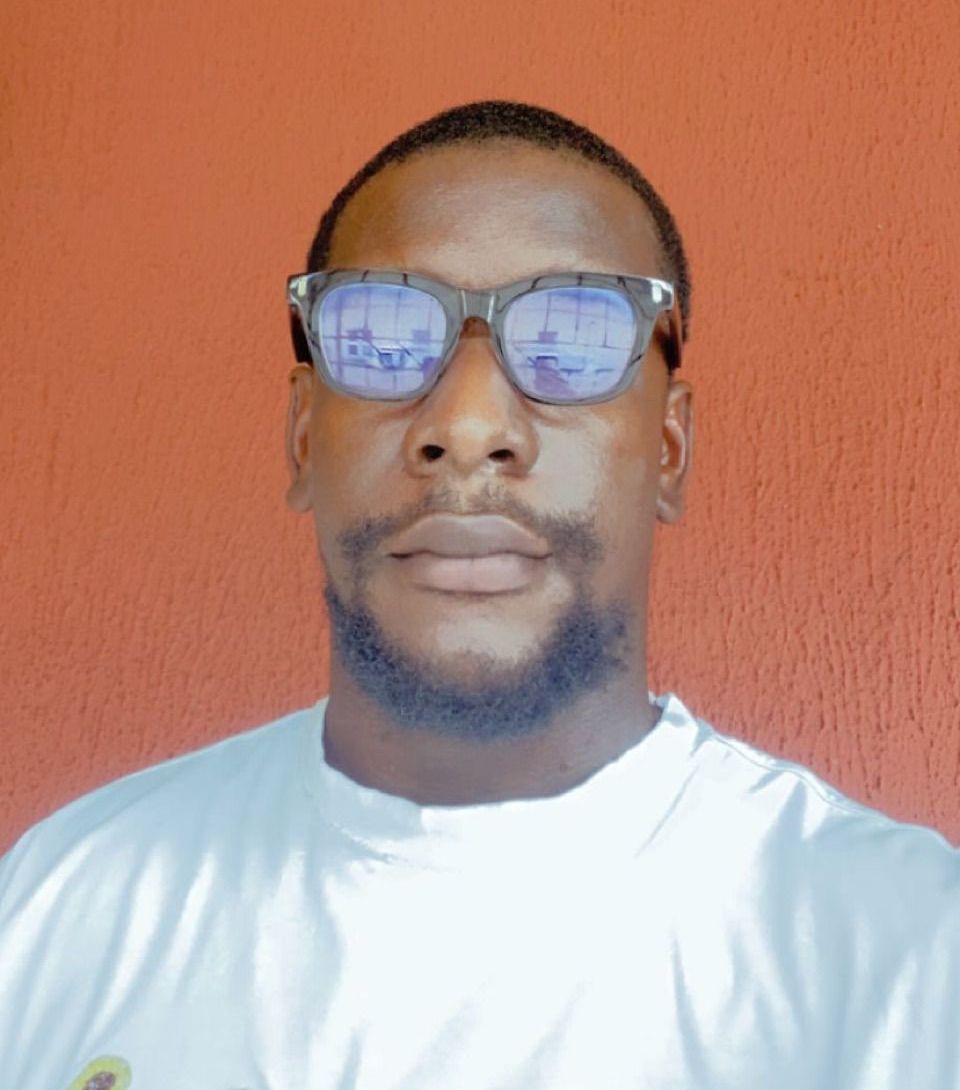
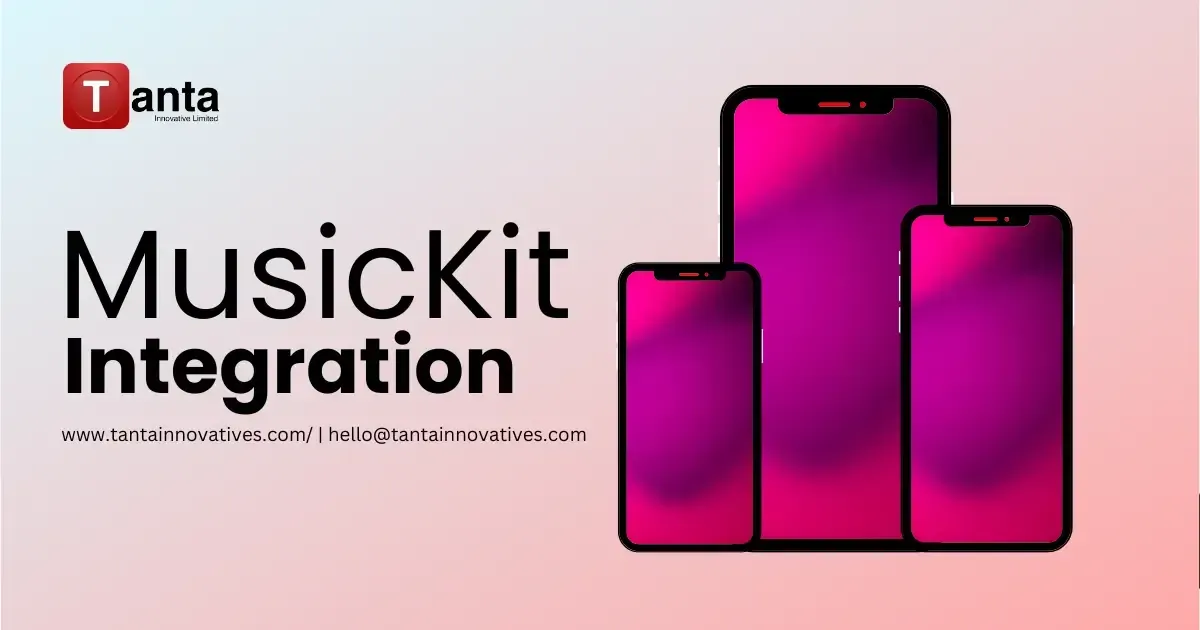
Mastering MusicKit Integration in iOS Applications
Learn how to integrate Apple Music features into your iOS app using MusicKit. Access a vast music library, enable seamle...
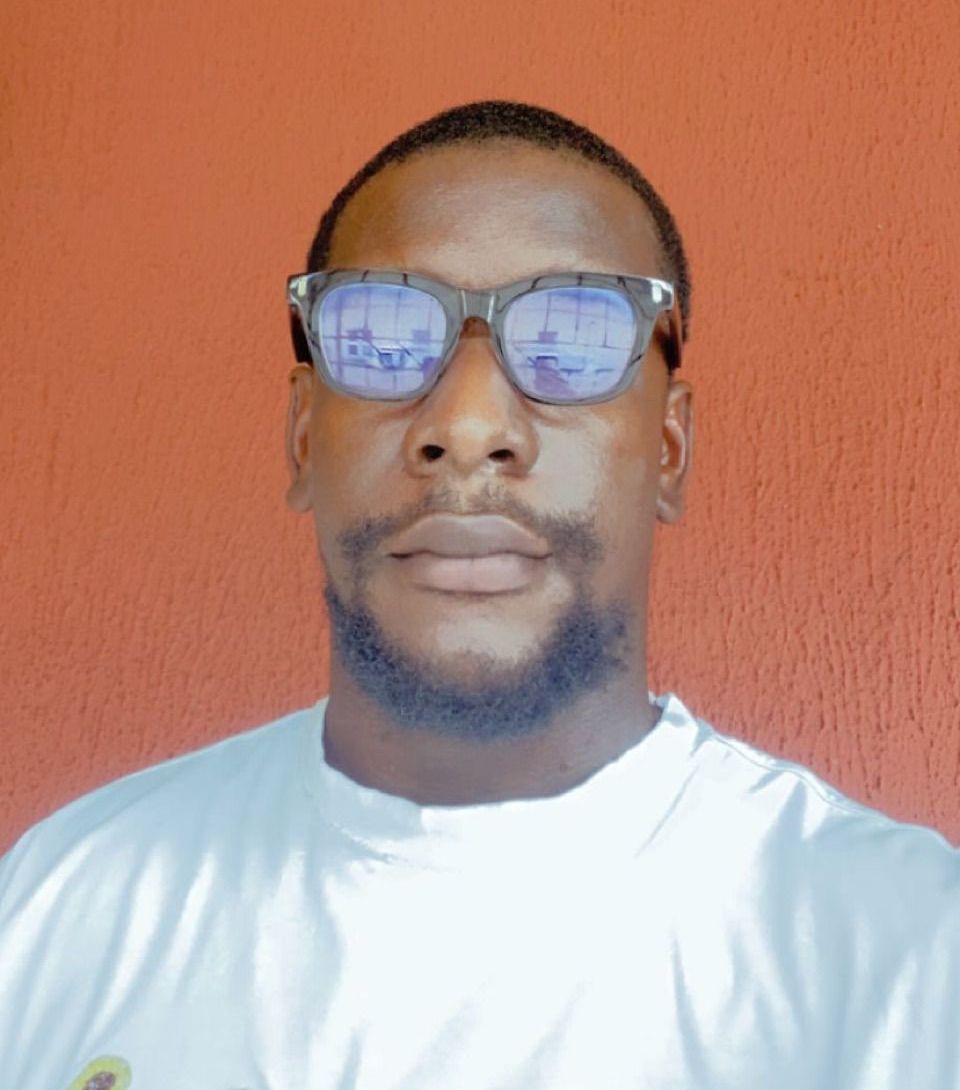
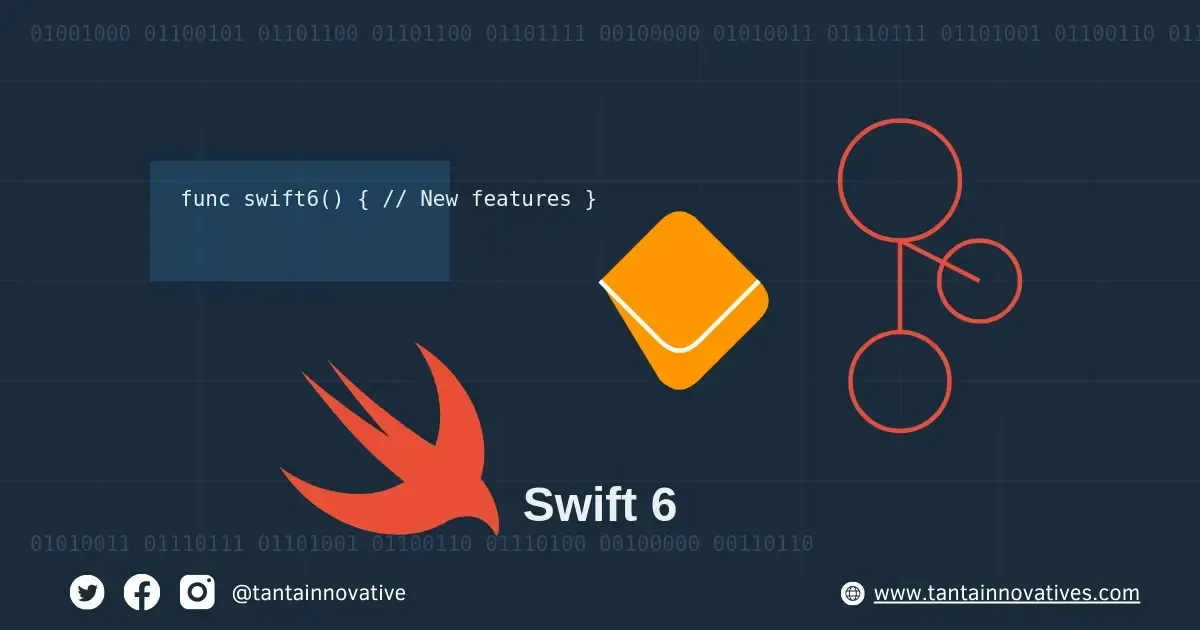
Introducing Swift 6 with Enhanced Concurrency and Performance
Swift 6 is here with groundbreaking updates, from improved concurrency and error handling to C++ interoperability and em...
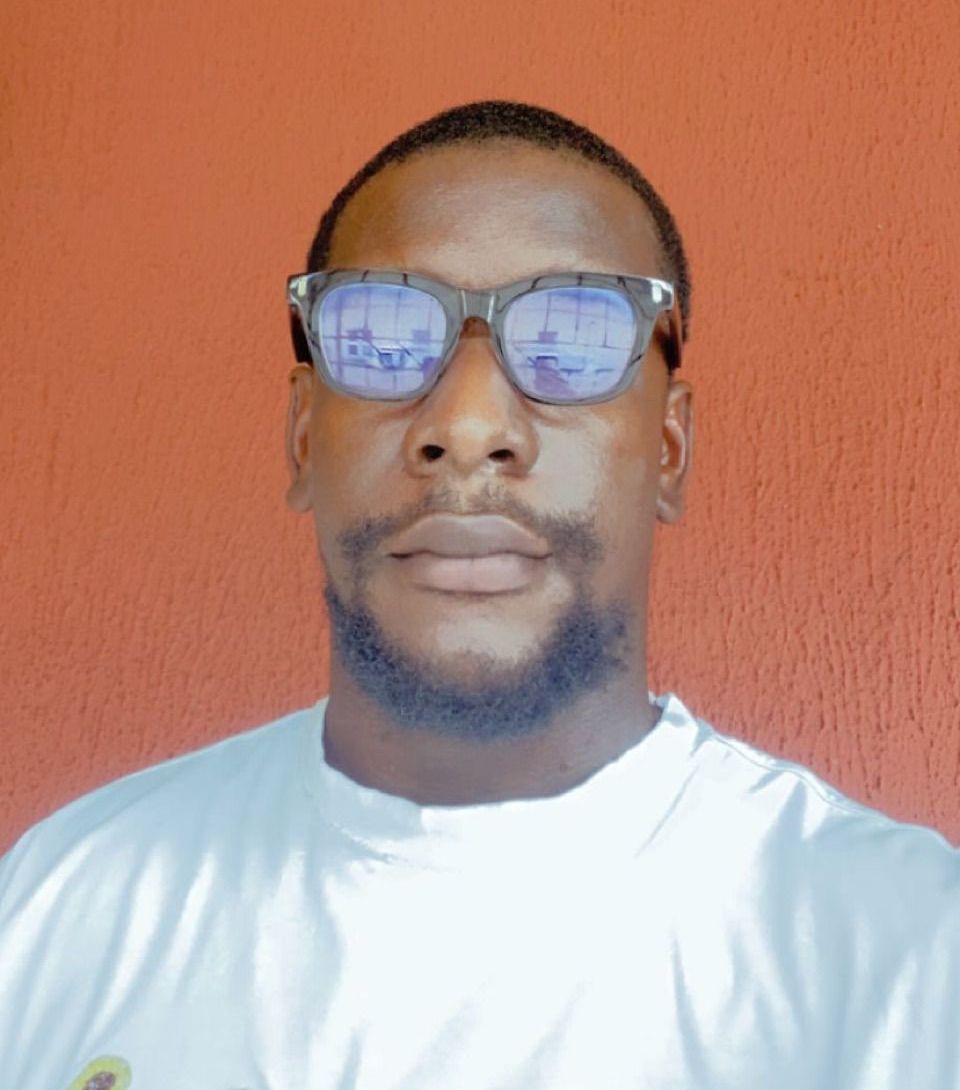

Setting Up Notifications in Xcode Cloud
In the fast-paced world of iOS development, staying informed about your build statuses is crucial. Xcode Cloud offers a ...
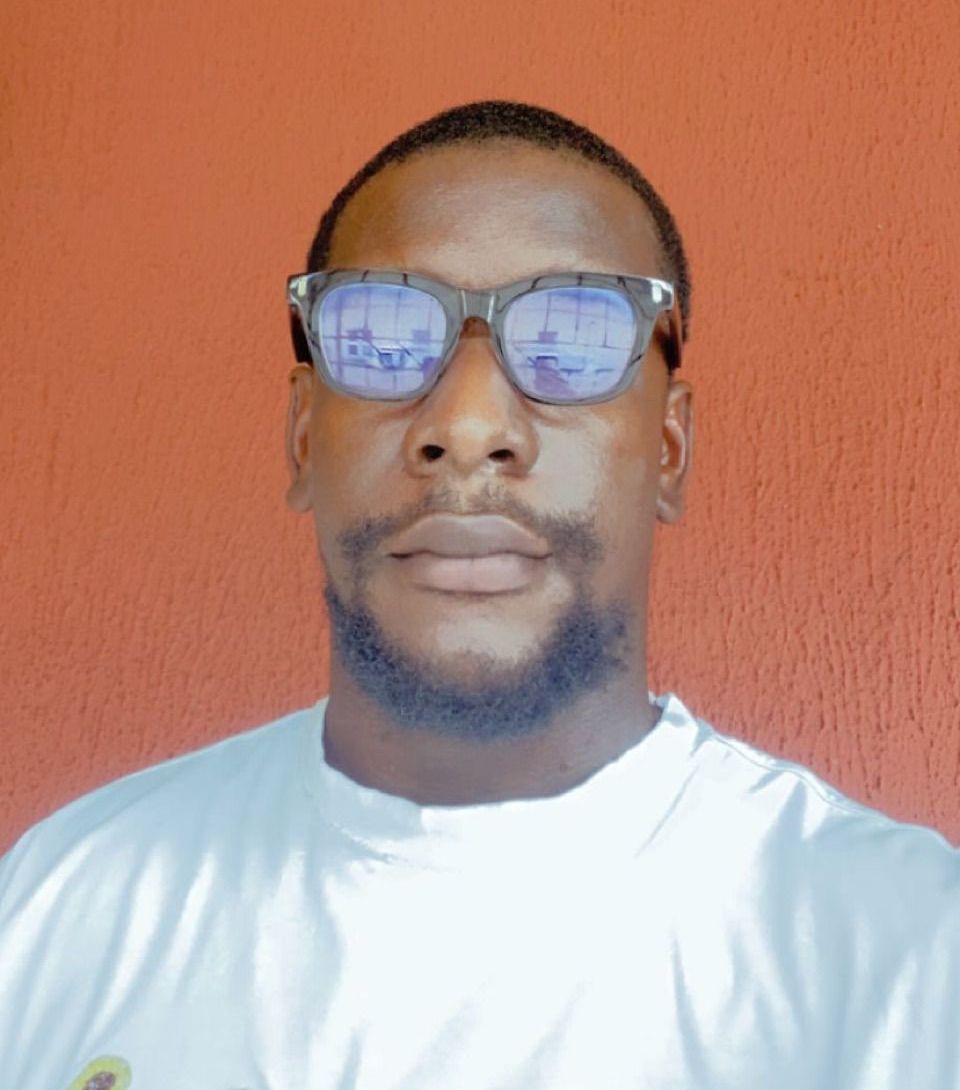