Getting Started with Google Maps in Flutter
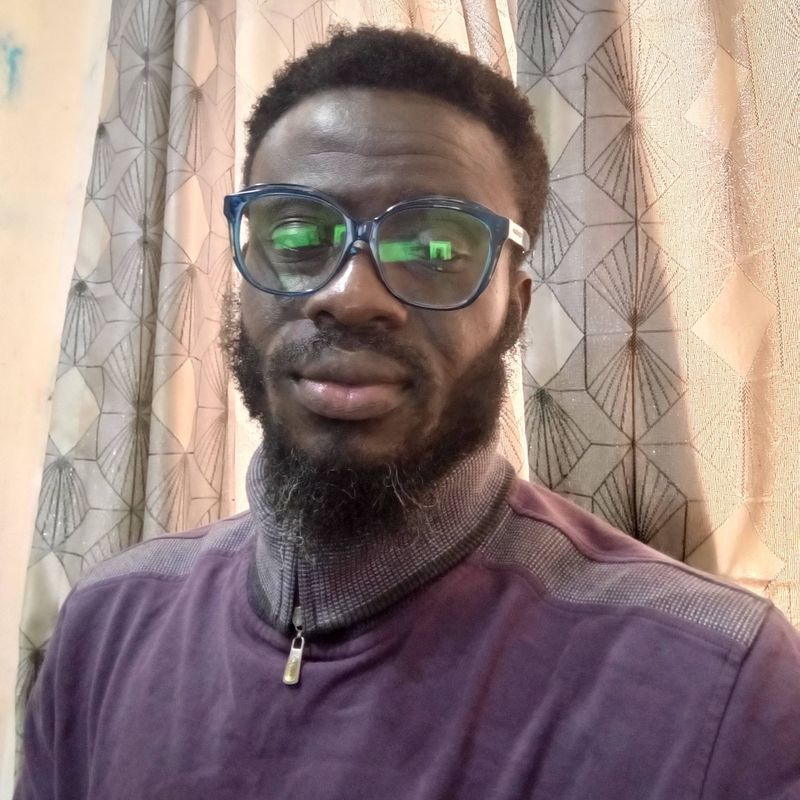
Omokehinde Igbekoyi
· 2 min read
0
0
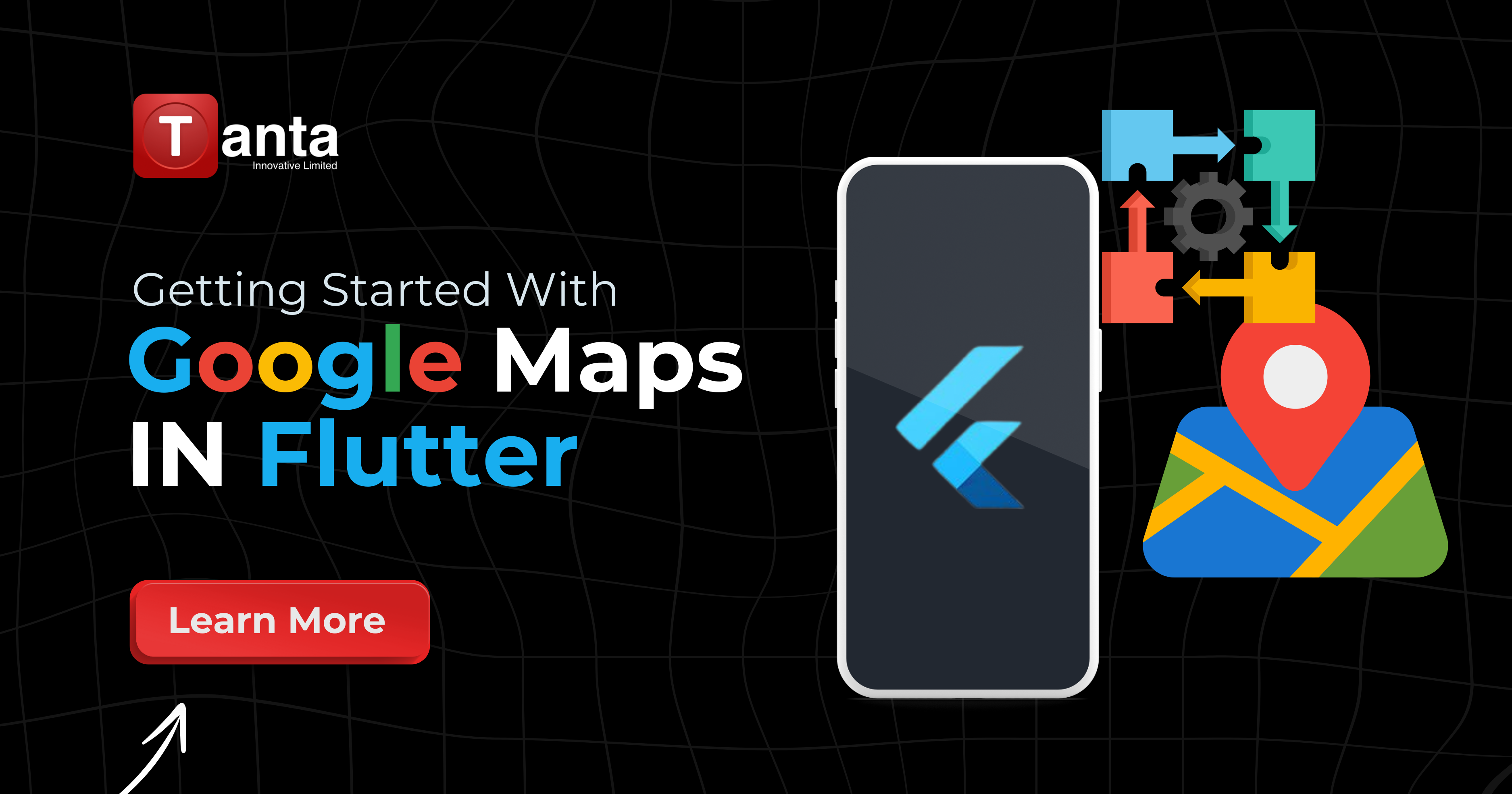
Hey there, Flutter developer! Ready to add some awesome maps to your app? I'll walk you through setting up Google Maps in your Flutter project, step by step. Don't worry - it might look tricky at first, but I'll make it super simple to follow along!
What We'll Cover
- Getting your Google Maps API key
- Setting up your Flutter project
- Adding the necessary configurations
- Creating your first map!
1. Getting Your Google Maps API Key
First things first - we need to get you an API key from Google. Here's how:
- Head over to the Google Cloud Console
- Create a new project (or select an existing one)
- Enable the Maps SDK for Android and iOS
- Create credentials (API key)
- Copy your new API key - we'll need it soon!
Pro Tip: Keep your API key safe and never share it publicly!
2. Setting Up Your Flutter Project
Now, let's get your Flutter project ready. Open your pubspec.yaml file and add the Google Maps package:
1dependencies:
2 flutter:
3 sdk: flutter
4 google_maps_flutter: ^2.5.0 # Latest version as of writing
Run flutter pub get to install the package.
3. Platform-Specific Setup
Android Setup
- Open android/app/src/main/AndroidManifest.xml
- Add this permission before the <application> tag:
1<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
- Add your API key within the <application> tag:
1<meta-data
2 android:name="com.google.android.geo.API_KEY"
3 android:value="YOUR-API-KEY-HERE"/>
iOS Setup
- Open ios/Runner/AppDelegate.swift (create it if it doesn't exist)
- Add this code:
swift
Copy
1import UIKit
2import Flutter
3import GoogleMaps
4
5@UIApplicationMain
6@objc class AppDelegate: FlutterAppDelegate {
7 override func application(
8 _ application: UIApplication,
9 didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
10 ) -> Bool {
11 GMSServices.provideAPIKey("YOUR-API-KEY-HERE")
12 GeneratedPluginRegistrant.register(with: self)
13 return super.application(application, didFinishLaunchingWithOptions: launchOptions)
14 }
15}
- Open ios/Runner/Info.plist and add:
1<key>io.flutter.embedded_views_preview</key>
2<true/>
4. Creating Your First Map!
Now for the fun part - let's create a basic map! Here's a simple example:
1import 'package:flutter/material.dart';
2import 'package:google_maps_flutter/google_maps_flutter.dart';
3
4class MapScreen extends StatefulWidget {
5 @override
6 _MapScreenState createState() => _MapScreenState();
7}
8
9class _MapScreenState extends State<MapScreen> {
10 GoogleMapController? mapController;
11
12 // Starting camera position
13 final LatLng _center = const LatLng(45.521563, -122.677433);
14
15 void _onMapCreated(GoogleMapController controller) {
16 mapController = controller;
17 }
18
19 @override
20 Widget build(BuildContext context) {
21 return Scaffold(
22 appBar: AppBar(
23 title: Text('My First Map!'),
24 ),
25 body: GoogleMap(
26 onMapCreated: _onMapCreated,
27 initialCameraPosition: CameraPosition(
28 target: _center,
29 zoom: 11.0,
30 ),
31 ),
32 );
33 }
34}
Common Issues and Solutions
- Black screen? Double-check your API key is correctly added in both platforms
- Map not showing? Make sure you have an active internet connection
- Permissions error? Verify you've added all required permissions in the manifest
What's Next?
Now that you've got your basic map working, you can:
- Add markers
- Draw polygons
- Implement custom map styles
- Add user location
- Create custom map controllers
Need Help?
If you run into any issues, check out:
- Stack Overflow with the tags [flutter] and [google-maps]
Happy mapping!