How to Integrate Intercom in Your Next.js 14 Project

Abraham Esandayinze Tanta
October 18, 2024 · 1 min read
0
0
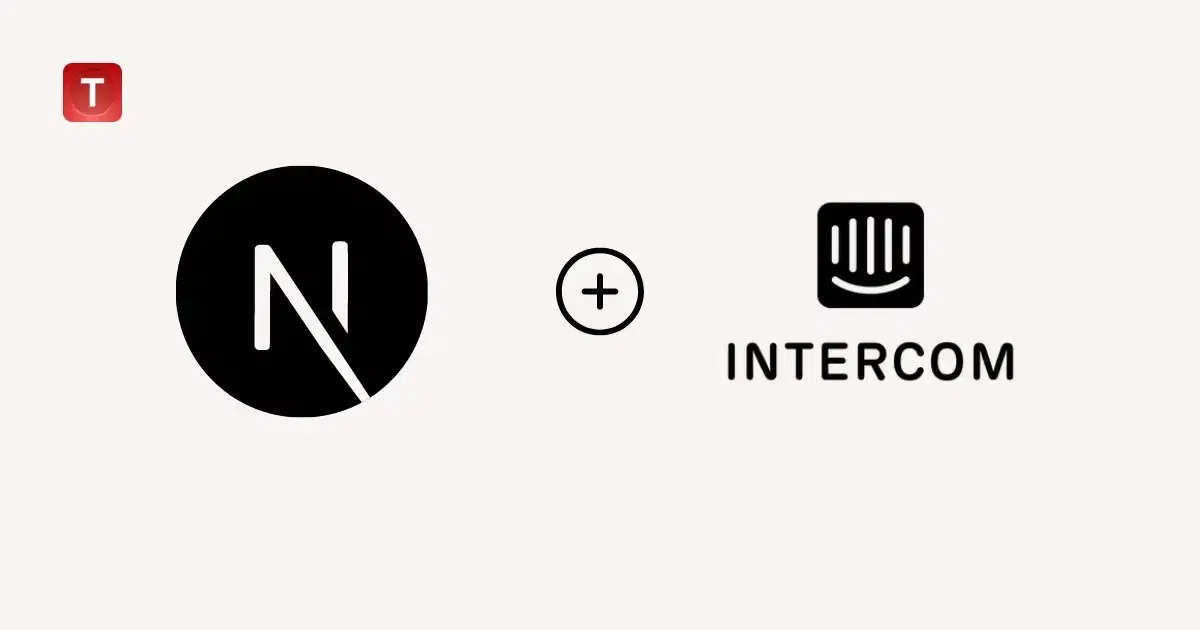
Intercom is a powerful tool for businesses needing efficient customer communication. Integrating it into your Next.js 14 project can enhance customer interactions and improve satisfaction.
Prerequisites
- An existing Next.js 14 project.
- Active Intercom account.
- Access to your Intercom app ID.
Step-by-Step Integration Guide
You’ll create a dedicated component for Intercom and integrate it into your layout. This will allow Intercom to load correctly throughout your project.
Step 1: Create IntercomClientComponent
- Go to your components folder.
- Create a file called IntercomClientComponent.tsx.
- Add this TypeScript code:
1// src/components/IntercomClientComponent.tsx
2
3'use client';
4import { useEffect } from "react";
5
6
7const IntercomClientComponent: React.FC = () => {
8 useEffect(() => {
9 window.intercomSettings = {
10 api_base: "https://api-iam.intercom.io",
11 app_id: "{YOUR_INTERCOM_APP_ID}" // Replace with your actual Intercom app ID.
12 };
13
14
15 if (window.Intercom) {
16 window.Intercom('reattach_activator');
17 window.Intercom('update', window.intercomSettings);
18 } else {
19 const script = document.createElement('script');
20 script.src = 'https://widget.intercom.io/widget/{YOUR_INTERCOM_APP_ID}';
21 script.async = true;
22 document.body.appendChild(script);
23 }
24 }, []);
25
26 return null;
27};
28
29export default IntercomClientComponent;
30
31
- Replace {YOUR_INTERCOM_APP_ID} with your Intercom app ID.
Step 2: Integrate into RootLayout
- Open your layout file (e.g., layout.tsx).
- Import the IntercomClientComponent.
- Add the Intercom script to ensure it loads after the page content.
1// src/layouts/RootLayout.tsx
2
3import React from "react";
4import Script from "next/script";
5import IntercomClientComponent from "@/components/IntercomClientComponent";
6
7const RootLayout = ({ children }: { children: React.ReactNode }) => (
8 <html lang="en">
9 <body>
10 <main>
11 {children}
12 </main>
13 <Script
14 strategy="afterInteractive"
15 id="intercom-settings"
16 dangerouslySetInnerHTML={{
17 __html: `
18 window.intercomSettings = {
19 api_base: "https://api-iam.intercom.io",
20 app_id: "{YOUR_INTERCOM_APP_ID}"
21 };
22 `,
23 }}
24 />
25 <IntercomClientComponent />
26 </body>
27 </html>
28);
29
30export default RootLayout;
31
32
33
Dynamic Integration of Intercom in Next.js 14
Pass user data to personalize customer interactions.
- Update IntercomClientComponent to accept user props:
1// src/components/IntercomClientComponent.tsx
2
3interface IntercomClientComponentProps {
4 userId?: string;
5 userEmail?: string;
6}
7
8const IntercomClientComponent: React.FC<IntercomClientComponentProps> = ({ userId, userEmail }) => {
9 useEffect(() => {
10 const settings = {
11 api_base: "https://api-iam.intercom.io",
12 app_id: "{YOUR_INTERCOM_APP_ID}",
13 user_id: userId,
14 email: userEmail,
15 };
16
17 window.intercomSettings = settings;
18
19 if (window.Intercom) {
20 window.Intercom('reattach_activator');
21 window.Intercom('update', settings);
22 } else {
23 const script = document.createElement('script');
24 script.src = 'https://widget.intercom.io/widget/{YOUR_INTERCOM_APP_ID}';
25 script.async = true;
26 document.body.appendChild(script);
27 }
28 }, [userId, userEmail]);
29
30 return null;
31};
32
33export default IntercomClientComponent;
- Pass user data from RootLayout:
<IntercomClientComponent
userId={user.id}
userEmail={user.email}
/>
Optimization Tips for Next.js 14 Script Management
- Use Next.js Script component to manage script loading for better performance.
- Set script loading to afterInteractive to prevent render-blocking issues.
Troubleshooting Common Integration Issues
- Script Errors: Ensure scripts load after all necessary DOM elements to avoid errors.
- User Data Not Updating: Confirm that props such as userId and userEmail are being correctly passed to the component.
Next Steps
- Visit our GitHub repository to contribute or explore more examples for integrating Intercom with Next.js 14.
- Stay updated with the latest improvements to both Intercom and Next.js for continuous enhancement of your customer engagement features.
As the Founder and CEO of Tanta Innovative Limited and Tanta Secure, I lead two IT firms that deliver innovative and secure solutions across Nigeria and beyond. With over a decade of expertise in ethical hacking, software development, Linux and network administration, I specialize in cybersecurity and malware detection. I hold a BSc in Computer Science from the Federal University of Technology and have earned multiple ethical hacking certifications. Fluent in Hausa, English, and French, I am passionate about leveraging the latest technologies to create value while ensuring the safety and privacy of users and their data.
More from Abraham Esandayinze Tanta
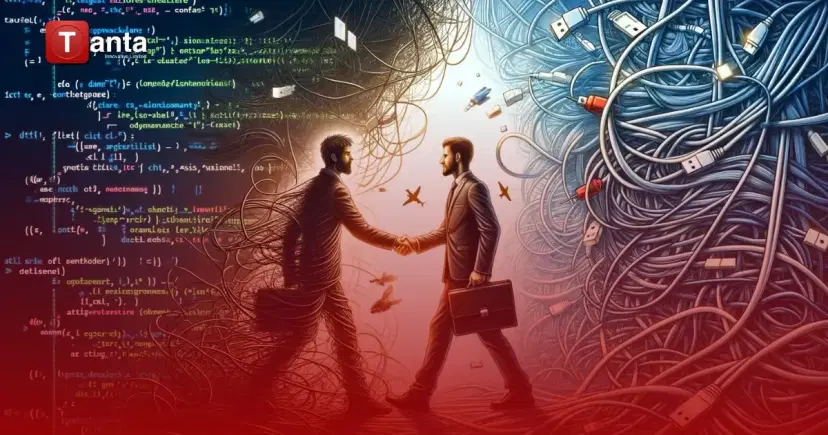
Guide to Choosing the Right Software Development Partner
Picking the right software development partner is vital for success. Asking the right questions helps you assess experti...

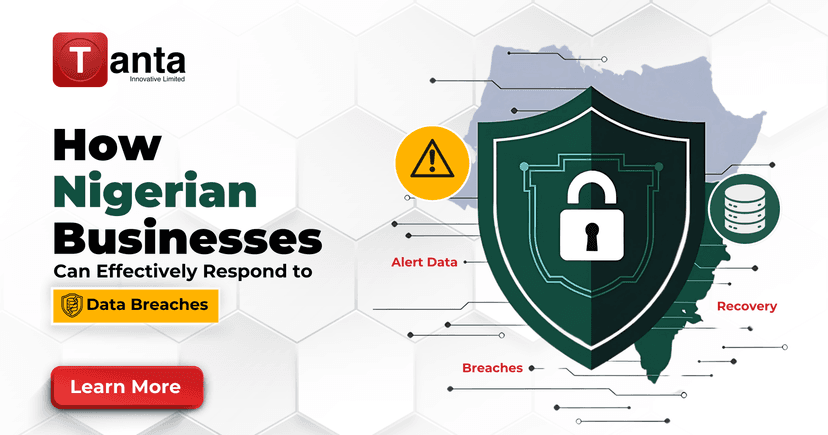
How Nigerian Businesses Can Effectively Respond to Data Breaches
Data breaches are a threat to any business. In Nigeria, the NDPR sets out strict guidelines that must be followed in th...


Cloud vs. On-Premise Software: Which is Right for Your Business?
As a CEO with 15 years of experience in Nigeria's IT sector, I've seen firsthand how the cloud vs. on-premise decision s...

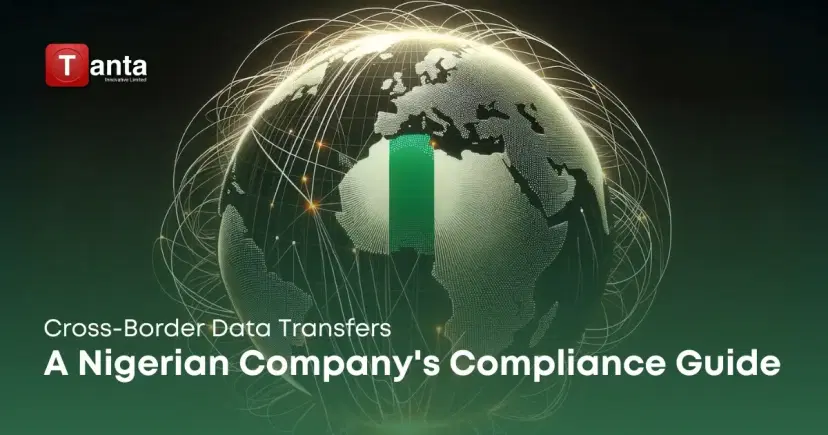
Cross-Border Data Transfers: A Nigerian Company's Guide to Global Data Compliance
Sending data across borders? Don't risk hefty fines and reputational damage. Learn how to safeguard your business in a g...
